10. 影像的幾何變換#
10.1. 裁切、調整大小和縮放影像#
影像為 NumPy 陣列(如影像 NumPy 快速入門章節所述),因此可以使用簡單的切片操作來裁切影像。以下我們裁切一個 100x100 的正方形,對應於太空人影像的左上角。請注意,此操作是對所有色彩通道進行的(色彩維度是最後的,第三維度)。
>>> import skimage as ski
>>> img = ski.data.astronaut()
>>> top_left = img[:100, :100]
為了改變影像的形狀,skimage.color
提供了幾個在縮放、調整大小和縮小中描述的函數。
from skimage import data, color
from skimage.transform import rescale, resize, downscale_local_mean
image = color.rgb2gray(data.astronaut())
image_rescaled = rescale(image, 0.25, anti_aliasing=False)
image_resized = resize(
image, (image.shape[0] // 4, image.shape[1] // 4), anti_aliasing=True
)
image_downscaled = downscale_local_mean(image, (4, 3))
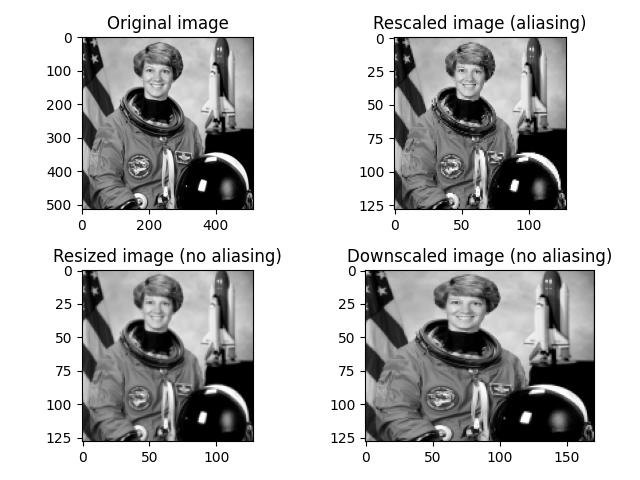
10.2. 投影變換(單應性)#
單應性是保留點對齊的歐幾里得空間變換。單應性的特定情況對應於保留更多屬性,例如平行性(仿射變換)、形狀(相似變換)或距離(歐幾里得變換)。scikit-image 中可用的不同類型單應性在單應性類型中介紹。
可以使用顯式參數(例如,縮放、剪切、旋轉和平移)來建立投影變換
import numpy as np
import skimage as ski
tform = ski.transform.EuclideanTransform(
rotation=np.pi / 12.,
translation = (100, -20)
)
或完整的變換矩陣
matrix = np.array([[np.cos(np.pi/12), -np.sin(np.pi/12), 100],
[np.sin(np.pi/12), np.cos(np.pi/12), -20],
[0, 0, 1]])
tform = ski.transform.EuclideanTransform(matrix)
變換的變換矩陣可以作為其 tform.params
屬性使用。變換可以通過使用 @
矩陣乘法運算符將矩陣相乘來組合。
變換矩陣使用齊次座標,這是歐幾里得幾何中使用的笛卡爾座標到更一般的射影幾何的擴展。特別是,無限遠的點可以用有限的座標表示。
可以使用skimage.transform.warp()
將變換應用於影像
img = ski.util.img_as_float(ski.data.chelsea())
tf_img = ski.transform.warp(img, tform.inverse)

skimage.transform
中的不同變換都有一個 estimate
方法,以便從兩組點(來源和目的地)估計變換的參數,如使用幾何變換教學中說明
text = ski.data.text()
src = np.array([[0, 0], [0, 50], [300, 50], [300, 0]])
dst = np.array([[155, 15], [65, 40], [260, 130], [360, 95]])
tform3 = ski.transform.ProjectiveTransform()
tform3.estimate(src, dst)
warped = ski.transform.warp(text, tform3, output_shape=(50, 300))
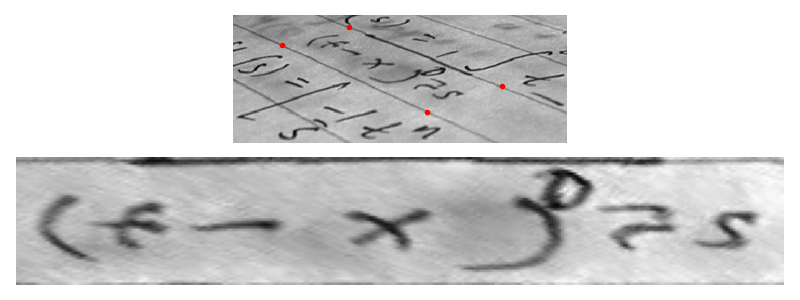
estimate
方法使用最小二乘最佳化來最小化來源和最佳化之間的距離。來源和目的地點可以手動確定,也可以使用skimage.feature
中可用的不同特徵偵測方法來確定,例如
角落偵測,
等等。
並使用skimage.feature.match_descriptors()
匹配點,然後再估計變換參數。但是,經常會產生虛假的匹配,建議使用 RANSAC 演算法(而不是簡單的最小二乘最佳化)來提高對離群值的穩健性,如使用 RANSAC 的穩健匹配中所說明。
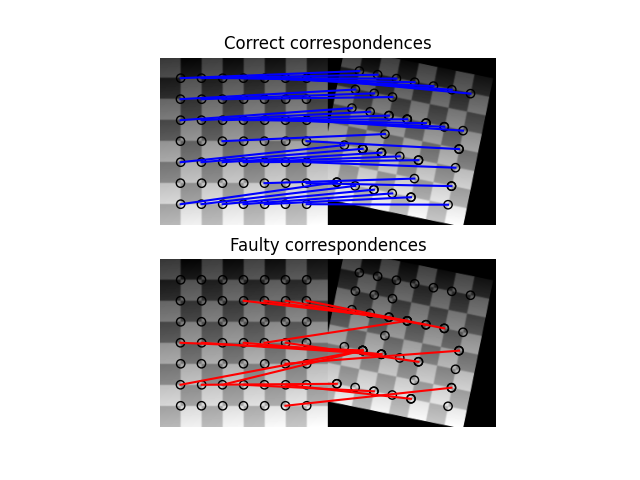
顯示變換估計應用範例有
estimate
方法是基於點的,也就是說,它僅使用來源和目標影像中的一組點。為了估計平移(位移),也可以使用基於傅立葉空間交叉相關的全域方法,此方法使用所有像素。此方法由 skimage.registration.phase_cross_correlation()
實現,並在影像配準教學中說明。
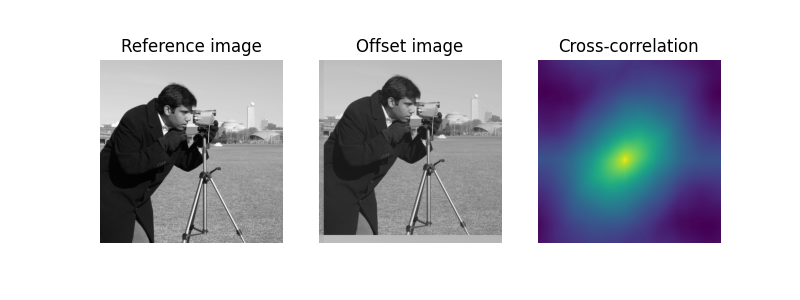
使用極座標和對數極座標變換進行配準教學解釋了此全域方法的一個變體,通過首先使用對數極座標變換來估計旋轉。