注意
前往結尾以下載完整的範例程式碼。或透過 Binder 在您的瀏覽器中執行此範例
將灰階濾鏡應用於 RGB 影像#
有許多濾鏡設計用於處理灰階影像,但不能處理彩色影像。為了簡化建立可適應 RGB 影像的函數的過程,scikit-image 提供了 adapt_rgb
裝飾器。
要實際使用 adapt_rgb
裝飾器,您必須決定如何調整 RGB 影像以用於灰階濾鏡。有兩個預定義的處理程式
each_channel
將每個 RGB 通道逐一傳遞給濾鏡,並將結果縫合回 RGB 影像。
hsv_value
將 RGB 影像轉換為 HSV,並將值通道傳遞給濾鏡。經過濾的結果會插入回 HSV 影像並轉換回 RGB。
以下,我們示範在幾個灰階濾鏡上使用 adapt_rgb
的方法
from skimage.color.adapt_rgb import adapt_rgb, each_channel, hsv_value
from skimage import filters
@adapt_rgb(each_channel)
def sobel_each(image):
return filters.sobel(image)
@adapt_rgb(hsv_value)
def sobel_hsv(image):
return filters.sobel(image)
我們可以像平常一樣使用這些函數,但現在它們可以同時處理灰階和彩色影像。讓我們用彩色影像繪製結果
from skimage import data
from skimage.exposure import rescale_intensity
import matplotlib.pyplot as plt
image = data.astronaut()
fig, (ax_each, ax_hsv) = plt.subplots(ncols=2, figsize=(14, 7))
# We use 1 - sobel_each(image) but this won't work if image is not normalized
ax_each.imshow(rescale_intensity(1 - sobel_each(image)))
ax_each.set_xticks([]), ax_each.set_yticks([])
ax_each.set_title("Sobel filter computed\n on individual RGB channels")
# We use 1 - sobel_hsv(image) but this won't work if image is not normalized
ax_hsv.imshow(rescale_intensity(1 - sobel_hsv(image)))
ax_hsv.set_xticks([]), ax_hsv.set_yticks([])
ax_hsv.set_title("Sobel filter computed\n on (V)alue converted image (HSV)")
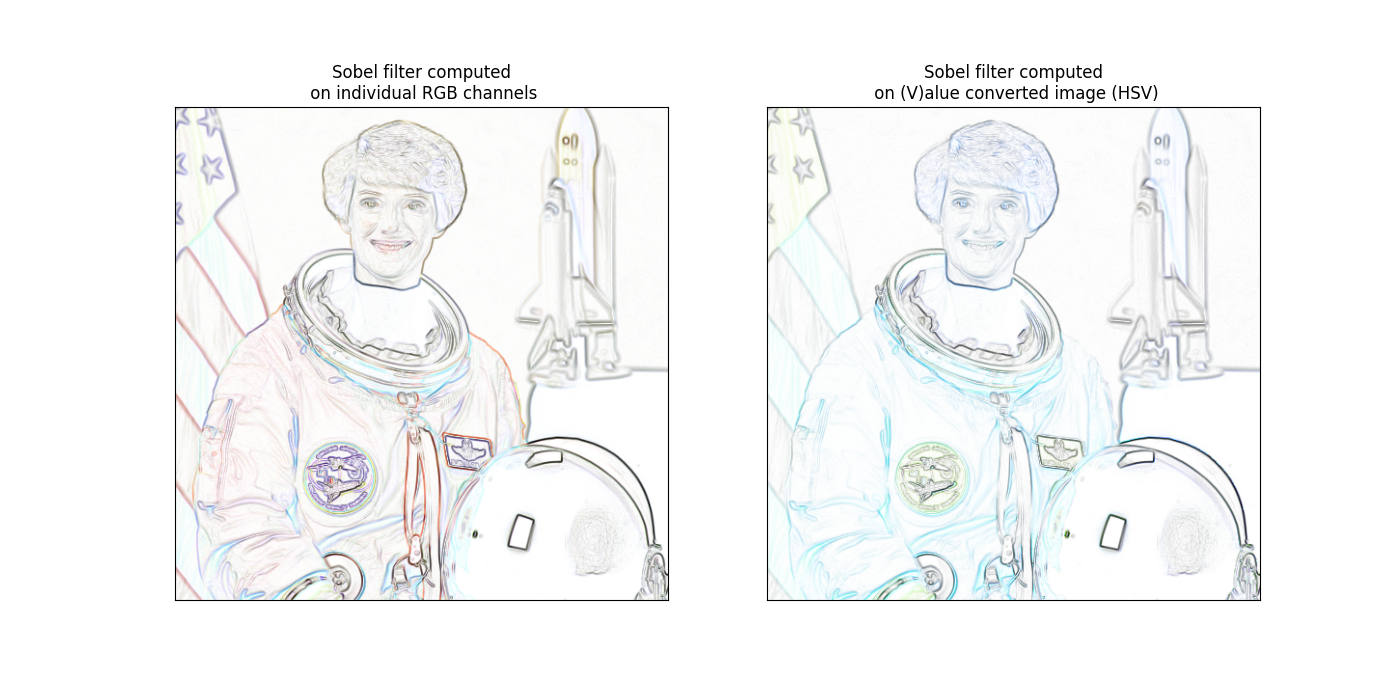
Text(0.5, 1.0, 'Sobel filter computed\n on (V)alue converted image (HSV)')
請注意,值過濾影像的結果保留了原始影像的色彩,但通道過濾影像的組合方式更令人驚訝。在其他常見情況下,例如平滑處理,通道過濾影像會比值過濾影像產生更好的結果。
您也可以為 adapt_rgb
建立自己的處理程式函數。若要這樣做,只需建立具有以下簽名的函數
請注意,adapt_rgb
處理程式是為影像作為第一個引數的濾鏡而撰寫的。
作為一個非常簡單的範例,我們可以將任何 RGB 影像轉換為灰階,然後傳回經過濾的結果
建立使用 *args
和 **kwargs
將引數傳遞給濾鏡的簽名很重要,這樣裝飾的函數才能有任意數量的位置和關鍵字引數。
最後,我們可以像以前一樣將此處理程式與 adapt_rgb
一起使用
@adapt_rgb(as_gray)
def sobel_gray(image):
return filters.sobel(image)
fig, ax = plt.subplots(ncols=1, nrows=1, figsize=(7, 7))
# We use 1 - sobel_gray(image) but this won't work if image is not normalized
ax.imshow(rescale_intensity(1 - sobel_gray(image)), cmap=plt.cm.gray)
ax.set_xticks([]), ax.set_yticks([])
ax.set_title("Sobel filter computed\n on the converted grayscale image")
plt.show()
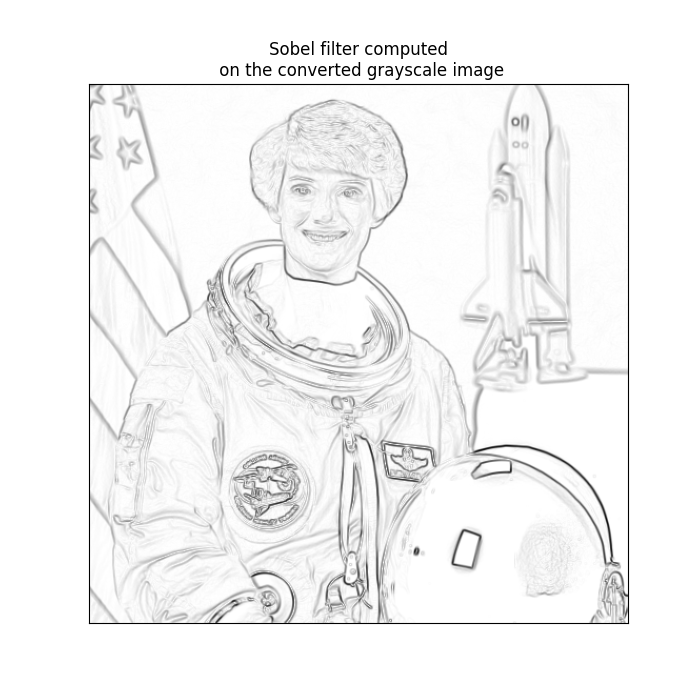
注意
使用一個非常簡單的陣列形狀檢查來偵測 RGB 影像,因此不建議對支援非 RGB 空間中的 3D 體積或彩色影像的函數使用 adapt_rgb
。
腳本的總執行時間: (0 分鐘 2.019 秒)